Introduction:
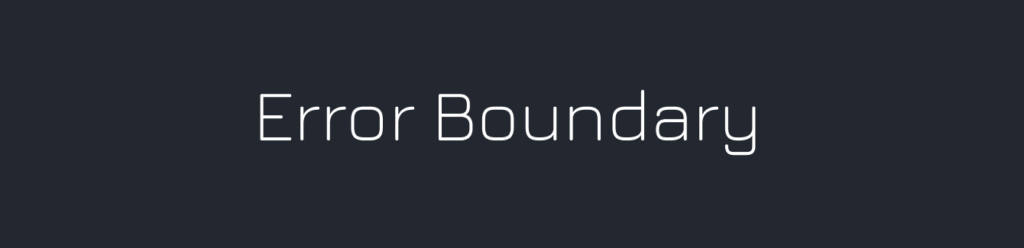
In React development, handling errors gracefully is essential for maintaining application stability and providing a smooth user experience. Errors can occur anywhere in your application, from rendering components to executing lifecycle methods, and if left unhandled, they can lead to crashes and poor user satisfaction. Enter Error Boundary, a powerful feature introduced by React to address this issue. In this extensive guide, we’ll explore Error Boundary in React, diving into their purpose, implementation, best practices, and real-world examples.
Understanding Error Boundary:
Error Boundaries are special React components that serve as a safety net for your application. They catch JavaScript errors that occur during the rendering of components, in lifecycle methods, or in the constructor of any child component within their subtree. By encapsulating potential error-prone components, Error Boundary prevents these errors from propagating up the component tree and crashing the entire application.
Why Use Error Boundary?
- Prevent Crashes: Error Boundary isolates errors to specific components, preventing them from propagating and crashing the entire application.
- Improve User Experience: Instead of displaying a blank screen or an intimidating error message, Error Boundary allows you to show a fallback UI, providing a more user-friendly experience.
- Debugging: Error Boundary log errors, making it easier to identify and debug issues in your application.
- Isolation: Errors occurring in one part of the application are contained by Error Boundaries, ensuring that they don’t impact other parts of the UI.
Implementing Error Boundaries:
To implement Error Boundaries in your React application, you need to create a new component that extends the React.Component class and define two lifecycle methods: componentDidCatch() and render(). Let’s take a closer look at how to do this.
Example:
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
componentDidCatch(error, errorInfo) {
// Log the error to an error reporting service
console.error('Error caught by ErrorBoundary:', error, errorInfo);
this.setState({ hasError: true });
}
render() {
if (this.state.hasError) {
// Fallback UI when an error occurs
return <h1>Something went wrong. Please try again later.</h1>;
}
return this.props.children;
}
}
export default ErrorBoundary;
In this example, we define an ErrorBoundary component that catches errors using the componentDidCatch() lifecycle method. If an error occurs within the ErrorBoundary’s child components, it updates its state to indicate that an error has occurred and displays a fallback UI instead of crashing the entire application.
Best Practices for Using Error Boundaries:
- Strategic Placement: Place Error Boundaries around components that you expect may fail, rather than wrapping the entire application.
- Keep Fallback UI Simple: The fallback UI displayed by Error Boundaries should be minimal and informative, avoiding complex logic or interactions.
- Thorough Testing: Test your Error Boundaries rigorously to ensure they handle errors gracefully and display the fallback UI correctly.
- Utilize Error Logging: Take advantage of error logging services to log errors caught by Error Boundaries, enabling you to track and debug issues effectively.
Real-World Examples:
To illustrate the power and versatility of Error Boundaries, let’s explore two real-world scenarios where they can be applied:
- Form Validation: Error Boundaries can be used to handle validation errors in forms, ensuring that invalid user input doesn’t crash the entire application.
- Third-Party Integrations: When integrating third-party libraries or APIs into your application, Error Boundaries can help isolate errors and provide a fallback UI in case of failures.
Conclusion:
Error Boundaries are a vital tool in your React developer toolkit, offering a robust mechanism for handling errors and ensuring application stability. By strategically implementing Error Boundaries and following best practices, you can build more reliable and resilient React applications that provide a seamless user experience even in the face of errors. Incorporate Error Boundaries into your development workflow and empower your applications with enhanced error-handling capabilities.
Developers also Love this: PURE COMPONENTS IN REACT