Introduction of Rest & Spread Operators in Javascript
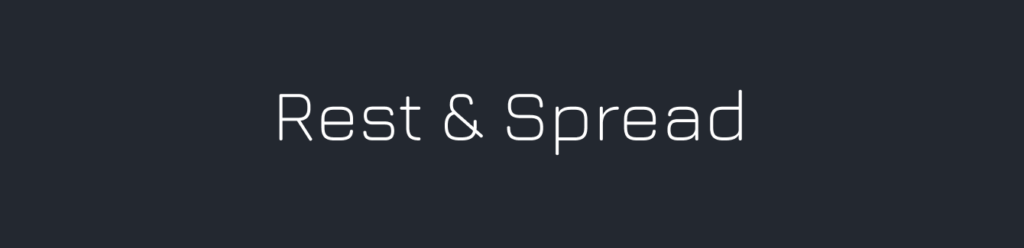
Rest and Spread Operators are powerful features introduced in ES6 (ECMAScript 2015) for working with arrays and objects in JavaScript. The rest operator (...
) allows us to represent an indefinite number of arguments as an array, while the spread operator also uses the ...
syntax to spread elements of an iterable (like an array) into places where multiple elements are expected.
Benefits
1. Simplify function parameter handling: The rest operator allows functions to accept any number of arguments without explicitly defining them.
2. Easily concatenate arrays: The spread operator makes it easy to concatenate arrays or add elements to an existing array.
3. Clone arrays and objects: Spread operators can be used to create shallow copies of arrays and objects, avoiding unwanted mutations.
4. Cleaner and more readable code: Rest & spread operators lead to more concise and expressive code, enhancing code readability and maintainability.
Comparison
While the Rest and Spread operators in Javascript share some similarities, they serve different purposes and are used in distinct contexts. The rest operator is primarily used in function definitions to gather function arguments into an array, while the spread operator is used in array literals, object literals, and function calls to expand elements or properties.
Another key difference is that the rest operator must be the last parameter in a function definition, whereas the spread operator can be used anywhere within an array or object literal. Additionally, the rest operator collects values into an array, while the spread operator spreads values out of an array or object.
In summary, both the Rest and Spread operators in Javascript are powerful features in JavaScript that contribute to cleaner, more concise code and improved developer productivity. By understanding their differences and use cases, developers can leverage these operators effectively to write more efficient and maintainable code.
How to Use?
Rest Operator ( … )
The Rest Operator ( … ) is used to capture all remaining arguments in a function or all remaining elements in an array.
- The rest operator is used to gather the remaining parameters into an array.
- It is represented by three dots (
...
) before the parameter name in a function definition. - It collects all the remaining arguments passed to a function into an array.
- It allows functions to accept an indefinite number of arguments.
- The rest parameter must be the last parameter in the function definition.
// Function to calculate sum of numbers
function sum(first, second, ...rest) {
let sum = first + second;
for(let i=0; i < rest.length; i++) {
sum += rest[i];
}
return sum;
}
console.log(sum(1, 2, 3, 4, 5)); // Output: 15
Spread Operator
The Spread Operator is used to spread the elements of an array or an object into a new array or object.
- The spread operator is used to expand elements of an array or properties of an object.
- It is represented by three dots (
...
) followed by the array or object that needs to be expanded. - It allows elements of an array to be expanded into another array or properties of an object to be copied into another object.
- It is commonly used for array concatenation, copying arrays, and object merging.
Here are examples of using the spread operator with both arrays and objects:
With Array:
const numbers = [1, 2, 3, 4, 5];
const moreNumbers = [6, 7, 8, 9, 10];
// Combining two arrays using the spread operator
const combinedNumbers = [...numbers, ...moreNumbers];
console.log(combinedNumbers); // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
With Object:
const person = { name: 'John', age: 30 };
const address = { city: 'New York', country: 'USA' };
// Combining two objects using the spread operator
const combinedPerson = { ...person, ...address };
console.log(combinedPerson);
// Output: { name: 'John', age: 30, city: 'New York', country: 'USA' }
In both examples, the spread operator (...
) expands the elements of the arrays or properties of the objects, allowing them to be combined into a new array or object respectively.
Conclusion
Rest and spread operators are invaluable tools for manipulating arrays and objects in JavaScript. They offer a concise and efficient way to work with collections and function arguments, leading to cleaner and more expressive code. By understanding and leveraging these operators, developers can write more robust and maintainable JavaScript applications.
Developer also Love this: 7 REASONS WHY REACT FRAGMENTS ARE ESSENTIAL IN MODERN DEVELOPMENT