Introduction to Pure Components
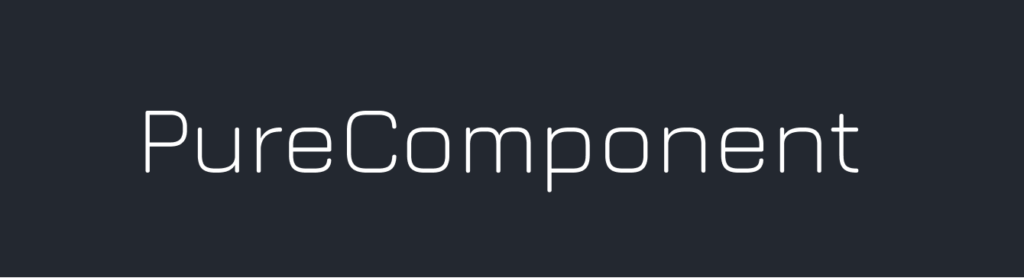
Let’s delve into the significance of PureComponents in modern development! As developers, optimizing rendering performance while maintaining code simplicity is paramount. PureComponents offers a compelling solution by minimizing unnecessary re-renders, thus enhancing the efficiency of React applications.
Understanding PureComponent
PureComponent in React.js are a specialized type of component designed to optimize rendering performance. Unlike regular components, Pure Component implements a shallow comparison of props and state to determine whether to re-render. This shallow comparison helps prevent unnecessary updates, resulting in improved rendering performance and reduced resource consumption.
Let’s explore some examples of implementing PureComponent in React.js for better understanding:
import React, { PureComponent } from 'react';
class MyPureComponent extends PureComponent {
render() {
return <div>{this.props.data}</div>;
}
}
In this example, MyPureComponent
extends PureComponent
instead of the standard Component
class. This ensures that the component will only re-render if its props or state change, leading to optimized performance.
Benefits of PureComponent
PureComponent offer several advantages over traditional components:
1. Performance Enhancement: By reducing the number of re-renders, PureComponent significantly improves the performance of React applications, especially those with complex UIs or large data sets.
2. Rendering Optimization: PureComponent leverage shallow comparisons to determine whether a re-render is necessary, leading to more efficient rendering and better overall responsiveness.
3. Preventing Unnecessary Renders: With PureComponent, React intelligently determines when a component needs to be updated, avoiding unnecessary renders and optimizing resource utilization.
4. Code Maintainability: By promoting a more declarative and efficient coding style, PureComponent contribute to code that is easier to understand, maintain, and debug.
Rendering Optimization with PureComponent
Rendering optimization is critical for ensuring optimal performance in React.js applications. Pure Components are a powerful tool in achieving this goal by minimizing unnecessary re-renders and improving overall rendering efficiency.
Pure Components are a specialized type of React component that implements a shallow comparison of props and state to determine whether the component should re-render. Unlike regular components, Pure Components only re-render when their props or state change, thereby avoiding unnecessary updates and improving performance.
Here are some key benefits and strategies for optimizing rendering with Pure Components:
- Automatic Memoization: Pure Components automatically perform memoization of props and state comparisons, eliminating the need for developers to manually implement memoization logic. This simplifies the development process and reduces the risk of introducing bugs related to memoization.
- Performance Improvement: By minimizing re-renders, Pure Components help improve the performance of React applications. This is especially beneficial in scenarios where components have complex render logic or are part of large component trees.
- Avoiding Wasted Renders: Regular components may re-render even when their props or state haven’t changed, leading to wasted rendering cycles. Pure Components prevent these unnecessary renders by performing shallow comparisons, ensuring that updates only occur when necessary.
- Better Scalability: As React applications grow in complexity, the number of components and their rendering frequency can impact performance. Pure Components help maintain performance scalability by reducing the rendering overhead associated with frequent updates.
- Enhanced User Experience: By optimizing rendering performance, Pure Components contribute to a smoother and more responsive user experience. Users will notice faster page load times, smoother transitions, and improved interaction responsiveness.
To leverage Pure Components effectively for rendering optimization, developers should:
- Identify components that would benefit from Pure Component optimization, especially those with frequent re-renders or complex render logic.
- Extend the
React.PureComponent
class for class-based components or use theReact.memo
higher-order component for functional components. - Ensure that the props passed to Pure Components are immutable or undergo shallow comparison to accurately determine changes.
- Monitor application performance using profiling tools to identify areas for further optimization and refinement.
Implementation Examples
Let’s explore some examples of implementing Pure Components in React.js:
import React, { PureComponent } from 'react';
class Counter extends PureComponent {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
incrementCount = () => {
this.setState(prevState => ({
count: prevState.count + 1
}));
};
render() {
return (
<div>
<h2>Counter: {this.state.count}</h2>
<button onClick={this.incrementCount}>Increment</button>
</div>
);
}
}
export default Counter;
In this example, we have a Counter
component that extends PureComponent
. It has a state property count
, which represents the current count value. The incrementCount
method is used to update the count value when the button is clicked.
Since Counter
extends PureComponent
, React automatically performs a shallow comparison of the component’s props and state whenever a render is triggered. If the props and state remain the same, the component will not re-render, thus optimizing rendering performance.
Conclusion
In conclusion, Pure Components play a crucial role in modern React development, offering significant performance benefits and enhancing code maintainability. By minimizing unnecessary re-renders and optimizing rendering efficiency, Pure Components empowers developers to create fast, responsive, and scalable React applications.
Embrace Pure Components in your React projects and experience the transformative impact on performance and user experience! 🚀✨
The Developers also love this:Â 7 REASONS WHY REACT FRAGMENTS ARE ESSENTIAL IN MODERN DEVELOPMENT
5 comments
My brother suggested I might like this website He was totally right This post actually made my day You cannt imagine just how much time I had spent for this information Thanks
I’m so glad to hear that you found the post helpful and that it made your day! It’s wonderful that your brother recommended the site to you. Knowing that the information was valuable and timely for you is very rewarding. Thank you for sharing your experience, and if there’s anything else you’re curious about, feel free to reach out.
Thank you for the auspicious writeup It in fact was a amusement account it Look advanced to far added agreeable from you However how can we communicate
Thanks for the appreciation, You can contact me on kevinitaliya123@gmail.com
Your article helped me a lot, is there any more related content? Thanks!